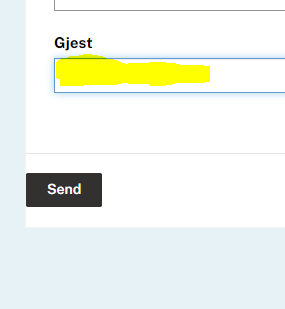
Auto fill form field with logged in user name
This case is narrowed down to minimal. The real form this example is taken from auto fills more user data than just the name.
However, with this example you hopefully understand the concept for broader use if you are new to it.
The case
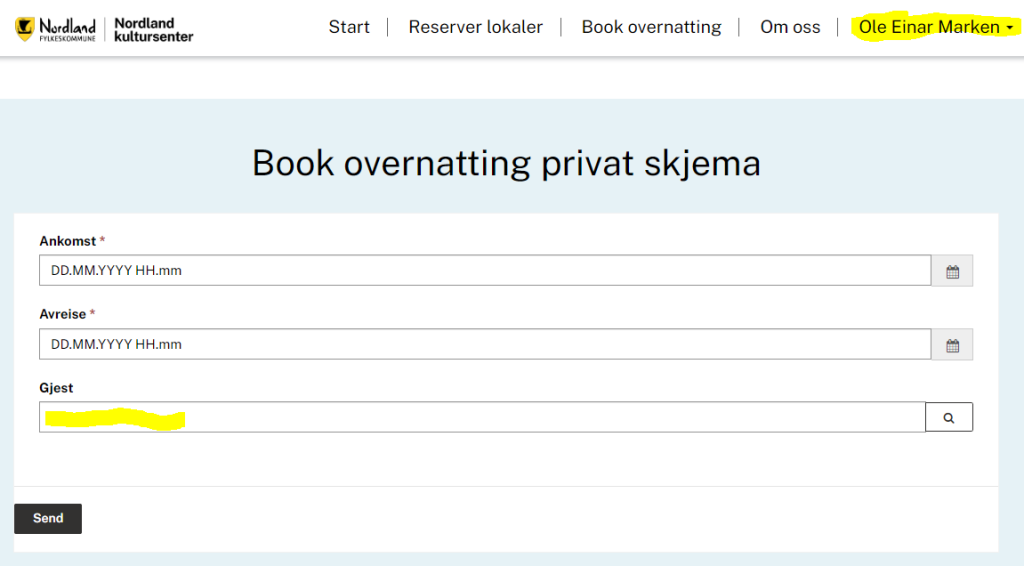
This part of a form for booking rooms asks the user for dates on arrival, departure, and the user’s name.
In the upper right corner we see the user is logged in. Instead of demanding the user to fill in the guest field manually (actually choosing himself from a lookup field), we want to fill in the name automatically.
The solution
We start examine the DOM for the lookup field. I use a web browser and hit F12. Then I select the field
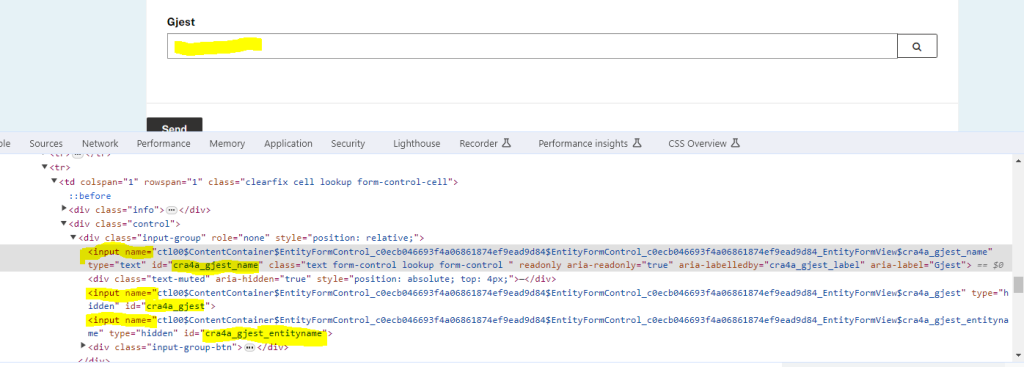
We see the input field we want to play with has three input tags, and each has it corresponding id which is related to our table where the user info relies.
PS! You sure also can get this info from your table field properties, for example via the power pages maker studio. In my opinion this browser console way is faster, but we might have different taste preferences regarding that.
We will need to add input values for those three inputs. I will use JQuery for that.
But first, lets have a look at which values are set if we choose the logged in user manually.
The form security settings are set so the users only can browse themselves in the lookup field.
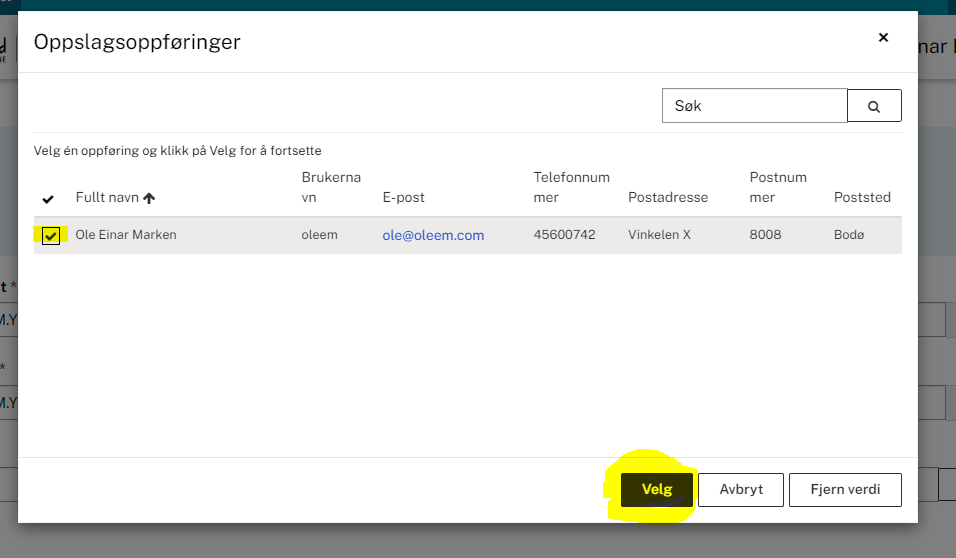
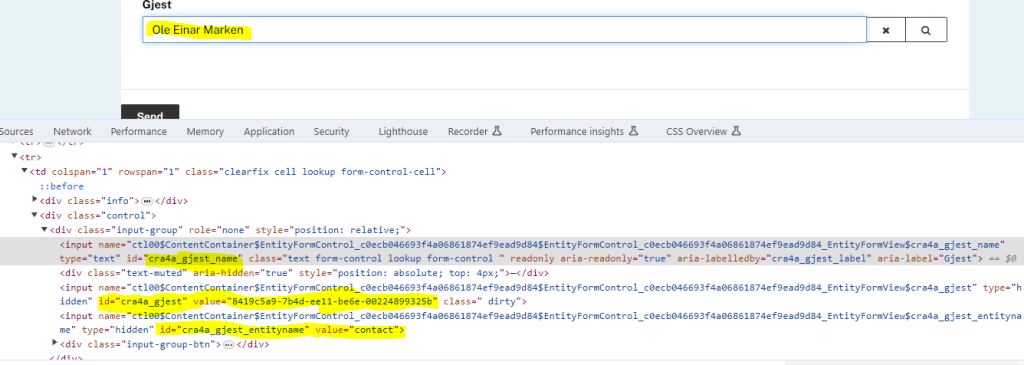
Now, look at the DOM which updates itself.
The first input has no new value set, visible in the DOM, but that doesn’t matter for our case.
The second input has set the value for the GUID for the field. This GUID will be different for each user.
The third input simply sets the value of the logical name for the table (entity) where the lookup field lives. Copy this value for further use. (Yes! Normally this will be contact as the users by default are stored there.)
Refresh the page, and then go to the code for the form. I prefer to open the VS Code in the browser from the maker studio. You go with what you prefer.
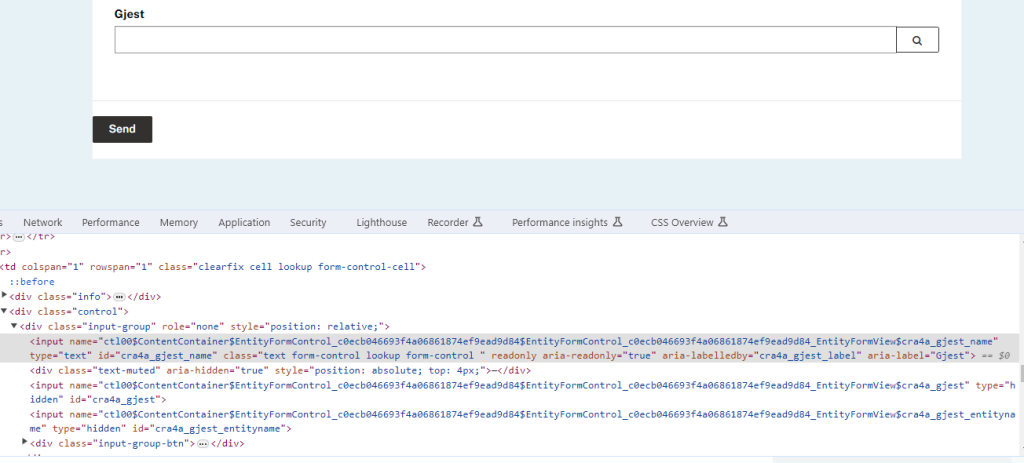
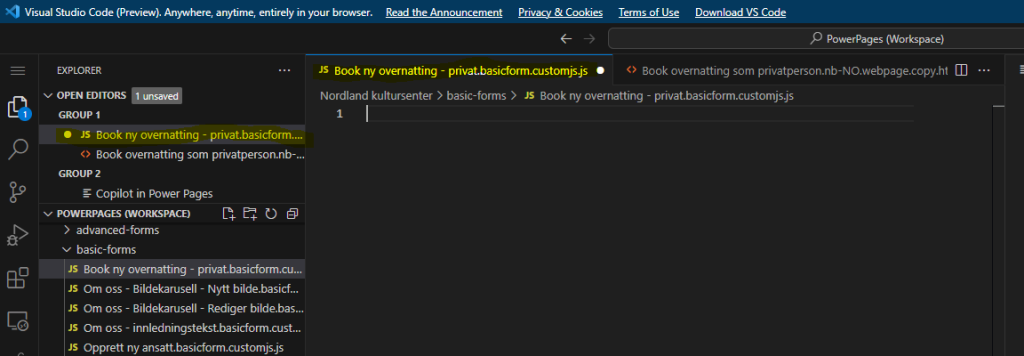
Writing our code…
We set up a function to run when the document is ready.
$(document).ready(function(){
});
We need to retrieve two values as variables.
For the first input with id = cra4a_gjest_name we just use liquid and the user object.to assign a variable for the logged in user’s full name.
var fullname = '{{ user.fullname }}'
For the second input id = cra4a_gjest we use the same method to assign the GUID to a variable for the logged in user.
var guid = '{{ user.id }}'
For the third input we already know, from previous steps, that the logical name for the table/entity is contact.
To set the values we add an attributes and assign the values to them. The name of the attributes will be value.
The complete code.
$(document).ready(function(){
var fullname = '{{ user.fullname }}'
var guid = '{{ user.id }}'
$("#cra4a_gjest_name").attr("value", fullname);
$("#cra4a_gjest").attr("value", guid);
$("#cra4a_gjest_entityname").attr("value","contact");
});
Save the code.
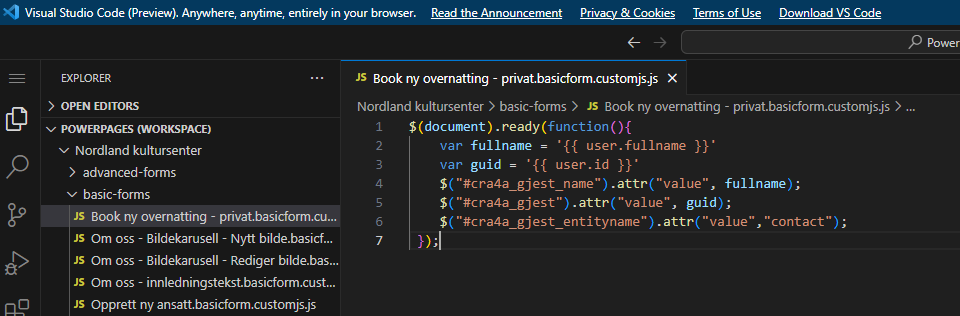
Refresh cache / synchronize. I use to do this from maker studio.
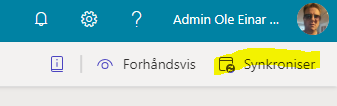
Refresh the page, and voila 🙂
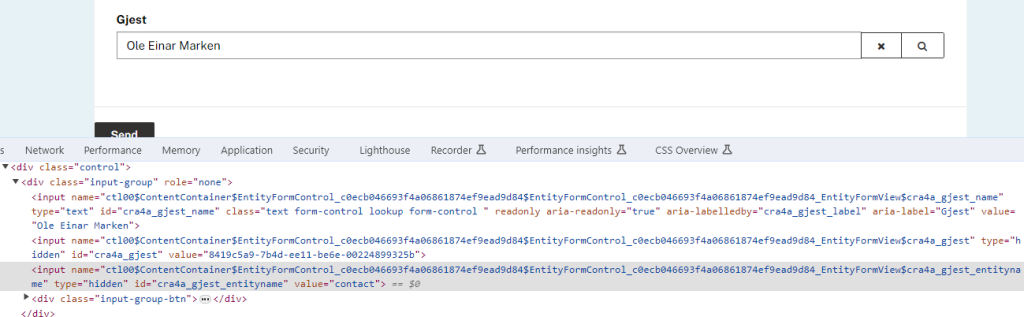
REMEMBER!
Be sure to test with different users – just in case.
Also test that your tables (and lists if you have any) are updated properly when you insert new records.
RELATED! Watch Tino Rabe’s video on how to do the same thing with multiple choise lookups